In the vast realm of Java programming, the Collections framework plays a pivotal role, providing a structured approach to handling and manipulating groups of objects. In this blog post, we’ll delve deep into the Collections Hierarchy in Java, unraveling its intricacies, and exploring the various interfaces and classes it encompasses.
Table of The Collections Hierarchy in Java
What is the Collections Hierarchy in Java?
At its core, the Collections Hierarchy in Java is a framework that provides a unified architecture for representing and manipulating collections of objects. These collections can be of various types, ranging from simple lists to complex sets and maps. The framework is designed to be both powerful and flexible, catering to the diverse needs of Java developers.
Key Interfaces in the Collections Hierarchy in Java
- Collection Interface: The root of the Collections Hierarchy, the
Collection
interface, serves as the foundation for all other interfaces. It defines the basic methods that all collections will support, such asadd
,remove
, andcontains
. - List Interface:Lists, ordered collections that allow duplicate elements, extend the
Collection
interface. TheList
interface introduces methods specific to sequential access and manipulation, such asget
,set
, andindexOf
. - Set Interface:For collections that do not allow duplicate elements, the
Set
interface is employed. It defines methods likeadd
andremove
, ensuring uniqueness within the collection. - Map Interface:The
Map
interface represents a collection of key-value pairs, where each key is associated with exactly one value. Methods likeput
,get
, andremove
facilitate efficient manipulation of mappings.
Why the Collections Hierarchy in Java Matters
Understanding the Collections Hierarchy is crucial for Java developers for several reasons:
- Code Reusability:By adhering to the interfaces defined in the Collections Hierarchy, developers can write code that is more generic and reusable. This promotes a modular approach to programming.
- Algorithm Efficiency:Different collections offer different time complexities for various operations. Knowing the hierarchy allows developers to choose the most suitable collection for a specific use case, optimizing algorithm efficiency.
- Interoperability:As various classes implement the common interfaces, collections become interoperable. This means that you can easily switch between different implementations without changing your code.
Details of Key Classes in the Collections Hierarchy in Java
- ArrayList:An implementation of the
List
interface,ArrayList
provides dynamic arrays that can grow as needed. Its constant-time access and variable-size nature make it a popular choice for many scenarios. - HashSet:As an implementation of the
Set
interface,HashSet
uses a hash table for storage. It provides constant-time performance for basic operations, making it an efficient choice for scenarios where uniqueness is crucial. - HashMap:Implementing the
Map
interface,HashMap
offers key-value pairs with constant-time performance for basic operations. It is widely used for its efficiency in mapping and retrieval.
Differences Between List and Set
Understanding the nuances between List
and Set
is vital for making informed design decisions in your Java applications.
List:
- Ordered Collection
- Allows Duplicate Elements
- Accessed by Index
- Examples:
ArrayList
,LinkedList
Set:
- Unordered Collection
- Does Not Allow Duplicate Elements
- No Index-based Access
- Examples:
HashSet
,TreeSet
Examples: Choosing the Right Collection
Consider a scenario where you need to store a list of tasks in a to-do application. In this case, using an ArrayList
would be appropriate, as it maintains the order of tasks and allows duplicates (tasks with the same name).
List<String> toDoList = new ArrayList<>();
toDoList.add("Complete Blog Post");
toDoList.add("Review Code");
toDoList.add("Attend Meeting");
On the other hand, if you are maintaining a set of unique user IDs, a HashSet
would be a more suitable choice.
Set<Integer> userIds = new HashSet<>();
userIds.add(101);
userIds.add(102);
userIds.add(103);
Chart: Collections Hierarchy Overview
Let’s visualize the Collections Hierarchy in Java in a chart:
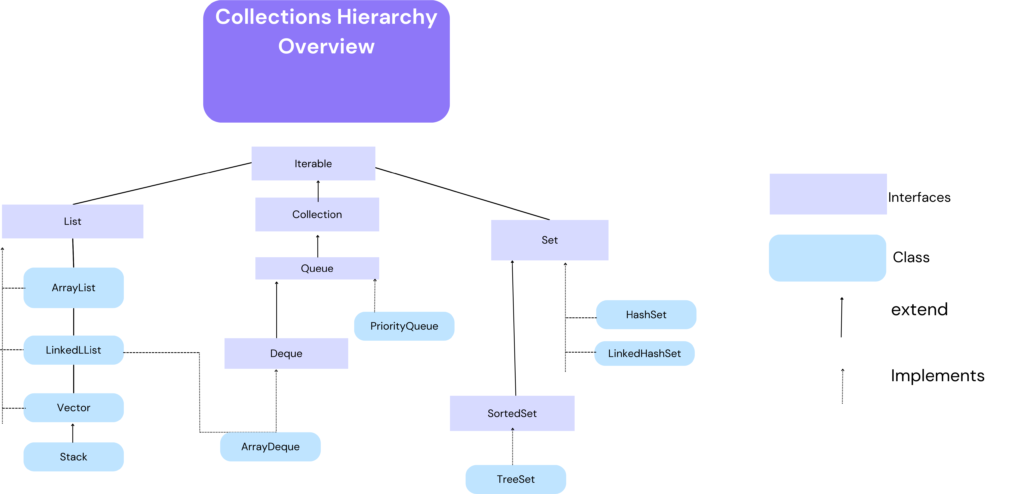
Conclusion
In conclusion, a solid understanding of the Collections Hierarchy in Java is fundamental for any Java developer. Whether you’re building simple to-do applications or complex enterprise systems, choosing the right collection can significantly impact performance and maintainability. By mastering the hierarchy and its nuances, you empower yourself to write efficient, scalable, and maintainable Java code.
By incorporating the Collections Hierarchy into your coding arsenal, you unlock the true potential of Java’s versatile Collections framework, paving the way for more robust and scalable applications. Happy coding!