Introduction
When it comes to concurrent programming in Java, understanding the life cycle of a thread is crucial. Threads are lightweight, independent processes that allow your Java applications to perform multiple tasks simultaneously. In this blog post, we’ll delve into the fascinating world of the “Java Thread Life Cycle,” providing a detailed overview and shedding light on the stages a thread goes through during its execution.
Table of the Java Thread Life Cycle
What is a Java Thread?
Before we explore the Java thread life cycle, let’s clarify what a Java thread is. A thread is the smallest unit of execution in a Java program. It represents an independent flow of control within a program. Threads enable you to perform multiple operations concurrently, which can significantly enhance the performance of your application.
Thread States
Threads in Java go through various states during their lifetime. Understanding these states is essential to grasp the Java thread life cycle. There are six primary thread states:
- New: This is the initial state when a thread is created but has not yet started. You can create a thread using the
Thread
class or by implementing theRunnable
interface and passing it to aThread
object. - Runnable: In this state, a thread is ready to run but is waiting for the CPU to execute it. Once the thread is scheduled by the Java Virtual Machine (JVM), it moves to the running state.
- Running: When a thread is executing its instructions, it’s in the running state. At any given time, only one thread can be in this state on a single CPU core.
- Blocked/Waiting: Threads in this state are temporarily inactive and not ready to run. They might be waiting for a resource, input, or other conditions to be met.
- Timed Waiting: A thread enters this state when it needs to wait for a specific period. This can be achieved using methods like
Thread.sleep()
or waiting on objects. - Terminated: The thread has completed its execution or has been explicitly terminated. Once a thread enters this state, it cannot return to any other state.
THE JAVA THREAD LIFE CYCLE DIAGRAM
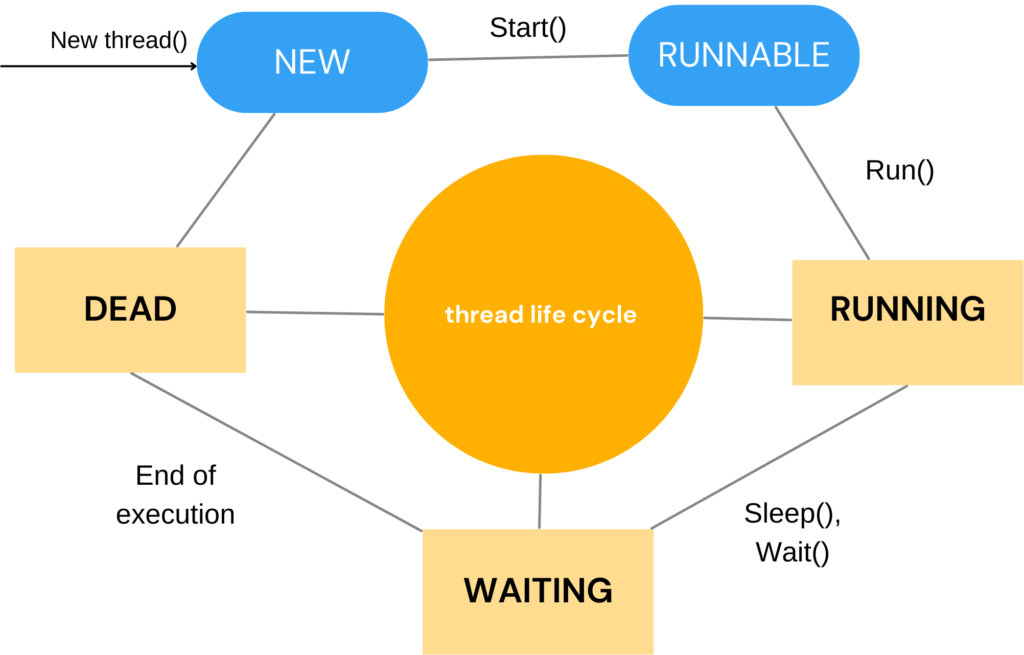
Thread Creation
To start the Java thread life cycle, you first need to create a thread. This can be accomplished in two ways in Java:
Extending the Thread Class
class MyThread extends Thread {
public void run() {
// Your thread's logic here
}
}
MyThread thread = new MyThread();
thread.start();
Implementing the Runnable Interface
class MyRunnable implements Runnable {
public void run() {
// Your thread's logic here
}
}
Thread thread = new Thread(new MyRunnable());
thread.start();
Both methods lead to the “New” state, as the thread has been created but hasn’t started its execution yet.
Starting and Running Threads
To transition a thread from the “New” state to the “Runnable” state, you need to call the start() method. The JVM takes care of scheduling the thread for execution. Once the thread is in the “Runnable” state, it competes with other threads for CPU time and can transition to the “Running” state.
Managing Thread Transitions
Thread Sleep
Sometimes, you may want a thread to pause for a specific duration. You can achieve this using the Thread.sleep() method. When a thread calls this method, it enters the “Timed Waiting” state for the specified duration and then returns to the “Runnable” state.
try {
Thread.sleep(1000); // Sleep for 1 second
} catch (InterruptedException e) {
// Handle the exception
}
Thread Join
The join() method allows a thread to wait until another thread completes. When a thread calls join() on another thread, it enters the “Blocked/Waiting” state until the target thread finishes its execution.
Thread t1 = new Thread(new MyRunnable());
Thread t2 = new Thread(new MyRunnable());
t1.start();
t2.start();
try {
t1.join(); // Wait for t1 to finish
t2.join(); // Wait for t2 to finish
} catch (InterruptedException e) {
// Handle the exception
}
Thread Synchronization
Thread synchronization is vital to prevent data races and ensure the threads behave as expected. This is typically achieved using keywords like synchronized or using locks such as ReentrantLock.
public class Counter {
private int count = 0;
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
}
By synchronizing methods or code blocks, you can control access to shared resources and prevent conflicts between threads.
Handling Thread Termination
Threads can terminate naturally when they reach the end of their run() method or explicitly using the stop() method. However, it’s considered bad practice to use stop() as it can lead to resource leaks and unpredictable behavior. The preferred way to end a thread is by letting it complete its execution naturally.
Conclusion
Understanding the Java Thread Life Cycle is crucial for developing concurrent applications in Java. Threads go through various states, from “New” to “Terminated,” and understanding how they transition between these states is essential for effective thread management. By visualizing the thread life cycle and using proper synchronization techniques, you can create robust and efficient multi-threaded applications.
In this blog post, we’ve covered the basics of the Java Thread Life Cycle, provided a visual representation of the thread states, and discussed important concepts like thread creation, starting and running threads, managing thread transitions, and handling thread termination. By mastering these concepts, you’ll be well-equipped to develop efficient and reliable Java applications with multi-threading capabilities.
Feel free to reach out if you have any questions or need further clarification on any aspect of the Java Thread Life Cycle. Happy coding!