Declaring arrays in Java is of paramount importance as it forms a cornerstone of Java programming. Arrays serve as a fundamental data structure, efficiently capable of storing and providing access to multiple values of identical data types. This article delves into the various facets of mastering the art of declaring arrays in Java, highlighting their significance and utility in the programming realm.
Contents for Declaring Arrays in Java
What is an Array?
Declaring arrays in Java involves creating a collection of elements sharing a common data type. This practice establishes a structured method for both arranging and handling data. Within arrays, indexed storage is employed, where each individual element is granted a distinct index, thus serving as its exclusive identifier within the array. This concept of indexed storage significantly enhances the efficiency of retrieving and manipulating array elements.
Unlike variables and objects, arrays can hold multiple values instead of just a single value. They offer a convenient way to manage and access related data collections.
Declaring Arrays in Java
For declaring arrays in Java, you need to follow a specific syntax. The declaration involves specifying the element type that the array will hold and providing a name for the array. Additionally, square brackets “[]” are used to indicate array creation.
For example, to declare an array of integers named “numbers”, you would write:
int[] numbers;
In some cases, you may dynamically allocate space for the array using the “new” keyword. This allows you to create arrays with sizes determined during runtime, rather than being fixed at compile time. Here’s an example:
int[] dynamicArray = new int[5]; // Creates an array with a size of 5
Array Initialisation
After declaring arrays in java, you may want to initialise it with values. Array initialisation can be done using literal values or by assigning variables to individual array elements.
To initialise an array with literal values, you can do:
int[] numbers = {60, 30, 13, 49, 88,4,35}; // Initialises the array with the values 60, 30, 13, 49, 88,4 and 35
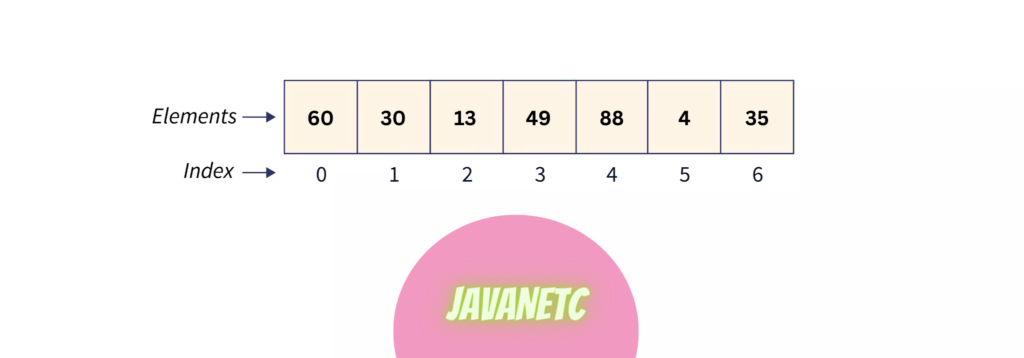
Alternatively, you can initialise an array with variables:
int[] dynamicArray = new int[3];
dynamicArray[0] = 10; // Assigns the value 10 to the first element
dynamicArray[1] = 20; // Assigns the value 20 to the second element
dynamicArray[2] = 30; // Assigns the value 30 to the third element
Array Sizes and Length
When declaring arrays in java, it’s essential to understand array sizes and their limits. The size of an array represents the number of elements it can hold and must be specified at the time of array creation.
To retrieve the length of an array, you can use the length attribute. For example:
int[] numbers = {1, 2, 3, 4, 5};
int arrayLength = numbers.length; // Retrieves the length of the array
Single-Dimensional Arrays
Single-dimensional arrays are the simplest form of arrays, consisting of a single row or sequence of elements. They are easy to understand and use. To declare and initialize a single-dimensional array, follow the discussed syntax.
You can access and modify elements in a single-dimensional array by using their respective indices. For instance:
int[] numbers = {1, 2, 3, 4, 5};
int firstElement = numbers[0]; // Accesses the first element of the array (1)
numbers[3] = 10; // Modifies the fourth element of the array (4) to 10
Multi-Dimensional Arrays
In addition to single-dimensional arrays, Java also supports multi-dimensional arrays. These arrays have multiple rows and columns, akin to a matrix-like structure. To declare and initialise a multi-dimensional array, use nested square brackets.
Accessing and modifying elements in multi-dimensional arrays follow a similar approach to single-dimensional arrays, but with an additional level of indexing to navigate through the dimensions. Multi-dimensional arrays are commonly used for representing matrices in mathematics or storing data in tables.
Arrays vs. ArrayList
Arrays and ArrayLists are both useful for managing collections of data, but they have distinct differences. Arrays have a fixed size determined at creation, whereas ArrayLists can dynamically grow and shrink as needed.
Arrays provide simplicity and efficiency, as they offer direct access to elements using index values. However, ArrayLists offer greater flexibility in terms of size adjustment and convenient methods for common operations like adding or removing elements.
If you have a fixed number of elements or require direct access, arrays may be a better choice. If you anticipate frequent size changes or need additional functionality, ArrayLists can be more suitable.
Common Mistakes to Avoid
When declaring arrays in Java, beginners often encounter common mistakes. To help you avoid these pitfalls, here are a few tips:
- Ensure index values are within the valid range of the array.
- Pay attention to proper array initialisation using literals or variables.
- Be cautious of null values to prevent NullPointerExceptions.
- Double-check array sizes to avoid overwriting neighbouring memory.
By keeping these tips in mind, you can minimize errors, improve the reliability of your array implementations, and adhere to best practices.
Advanced Array Techniques
Once you grasp the basics of declaring arrays in java, you can explore more advanced techniques to enhance your Java programming skills. This section highlights a few of these techniques:
- Anonymous arrays: These arrays are declared and instantiated without assigning them a variable name.
- Arrays of objects: You can create arrays that hold objects of a specific class or interface.
- Arrays of different data types: Java allows the creation of arrays that hold elements of different data types.
Object[] arr = new Object[6];
arr[0] = new String("First Pair");
arr[1] = new Integer(1);
arr[2] = new String("Second Pair");
arr[3] = new Integer(2);
arr[4] = new String("Third Pair");
arr[5] = new Integer(3);
By understanding and applying these advanced techniques, you can unlock the full potential of declaring arrays in java programs.
Passing and Returning Arrays to/from Methods
Java enables you to pass arrays to methods as parameters and return arrays from methods. This empowers you to perform various operations on arrays within methods and manipulate them as needed.
When passing arrays to methods, you can directly reference the array as an argument. Similarly, when returning arrays from methods, you can specify the return type as the array type.
By following best practices and examples, you can leverage the power of methods to process and transform your arrays effectively.
Sorting and Searching Arrays
Sorting and searching are common operations performed on arrays. Java provides various sorting algorithms like bubble sort, insertion sort, and quicksort, which can be implemented to sort arrays in ascending or descending order.
Similarly, you can apply searching algorithms like linear search or binary search to efficiently find specific elements within arrays.
By implementing these algorithms in Java, you can efficiently manage and locate elements within your arrays, improving performance and overall functionality.
Working with Arrays in Java Libraries
The Java language offers a rich ecosystem of libraries that provide additional functionality for working with arrays. For ex:- the java.util.Arrays class has several utility methods for manipulating arrays.
By exploring these built-in array methods and classes, you can extend the capabilities of your array-related operations and improve overall program efficiency.
Performance Considerations
When working with arrays, it’s important to consider performance implications. Analysing the time and space complexity of array operations can help optimize your code and ensure efficient memory usage.
By understanding array performance intricacies, you can make informed decisions about array size and structure, ultimately enhancing speed and reliability in your programs.
Exception Handling with Arrays
Exception handling is crucial when dealing with arrays to prevent unexpected runtime errors. One common exception when working with arrays is the IndexOutOfBoundsException, which occurs when attempting to access an element outside the valid range of indices.
To handle this exception, use try-catch blocks to catch any potential errors. Additionally, be aware of other potential exceptions that may arise when working with arrays and ensure appropriate error handling.
Summary
In conclusion, mastering the declaring arrays in java is a fundamental skill for every Java programmer. Arrays provide an efficient and structured way to manage collections of related data.
Throughout this article, we explored the concept of arrays, how to declaring arrays in java, different types of arrays, and their respective use cases. We also discussed advanced techniques, best practices, and common mistakes to avoid.
Solidify your understanding by practicing and experimenting with arrays in Java. Continuous improvement will make you a proficient Java developer, unlocking the vast possibilities arrays offer in programming.
FAQs
Here are some frequently asked questions related to array declaration in Java:
- What is the maximum size of an array in Java?
- How do I declare an array with an unknown size?
- How can I resize an array in Java?
- Can I have arrays of arrays in Java?
- How do I iterate over the elements of an array?
Feel free to explore these questions further as you continue your Java programming journey.
Conclusion
Declaring arrays in Java is a fundamental aspect of programming, enabling efficient organisation and manipulation of data. Through this article, we have covered essential concepts and techniques related to array declaration.
Understanding arrays, their syntax, initialisation methods, and various dimensions equips you to handle complex data structures effectively. By applying best practices, avoiding common mistakes, and exploring advanced techniques, you can master the art of array declaration in Java.
Remember that practice makes perfect. With diligent practice and deeper exploration, you will develop strong array usage and become a proficient Java programmer.