Introduction:
In Java, HashSet is a dynamic and unordered collection that provides efficient search operations by utilizing hashcodes. This article dives into the features, declaration, constructors, methods, and practical examples of HashSet in Java.
Table of Contents
Features of Java HashSet :
1.Unordered and Unsorted:
- HashSet does not maintain the order of insertion, as elements are organized based on their hashcode values.
2.Efficient Search Operations:
- Search operations are faster with an optimized hashCode() implementation, enhancing overall performance.
3.Unique Elements:
- HashSet ensures that only unique elements are stored, making it suitable for scenarios where duplicates are not allowed.
4.Null Value Handling:
- Allows only one null value to be stored.
5.Non-Synchronized:
- HashSet is non-synchronized, making it more efficient in scenarios without the need for thread safety.
6.Hash Table Data Structure:
- Internally, HashSet uses a hash table data structure to store elements based on their hashcodes.
Declaration of HashSet in Java:
// Syntax
HashSet<E> setName = new HashSet<E>();
// Example
HashSet<String> carData = new HashSet<String>();
HashSet in Java Example:
import java.util.*;
public class Main {
public static void main(String[] args) {
HashSet<String> carData = new HashSet<String>();
carData.add("Nano");
carData.add("Toyota");
carData.add("Volkswagen");
System.out.println(carData); // Output: [Nano, Volkswagen, Toyota]
}
}
Hierarchy and Constructors:
- HashSet in Java is a class in Java that extends the AbstractSet class and implements the Set interface.
- Constructors include default, capacity-based, load factor-based, and those initializing from a Collection.
Constructors of HashSet in Java:
- HashSet():
- Default constructor initializing a HashSet object with default capacity and load factor.
- HashSet(int capacity):
- Constructs a HashSet with a capacity equal to the specified argument. The HashSet can dynamically grow as more elements are added.
- HashSet(int capacity, int loadFactor):
- Creates a HashSet with a specified capacity and load factor, allowing customization of the initial size and resizing behavior.
- HashSet(Collection c):
- Constructs a HashSet and initializes it with elements from the provided Collection
c
, allowing seamless creation from existing collections.
- Constructs a HashSet and initializes it with elements from the provided Collection
Methods of HashSet in Java:
add(E e)
, remove(Object e)
, clear()
, clone()
, contains(Object e)
, isEmpty()
, iterator()
, size()
Modifier | Method Name | Description of the Method | Time Complexity |
---|---|---|---|
boolean | add(E e) | Adds an element of type E if not already present in HashSet | O(1) |
void | clear() | Clears the entire HashSet by removing all elements | O(1) |
Object | clone() | Creates and returns a shallow copy of the HashSet | O(N) |
boolean | contains(Object e) | Returns true if the specified element is present, false otherwise | O(1) |
boolean | isEmpty() | Returns true if the HashSet is empty, otherwise false | O(1) |
Iterator<E> | iterator() | Returns an iterator over all values present in the HashSet | O(N) |
boolean | remove(Object e) | Removes the specified element if present in the HashSet | O(1) |
int | size() | Returns the number of elements present in the HashSet | O(1) |
Examples of HashSet Operations:
1. Iterating Through HashSet:
HashSet<Student> studentData = new HashSet<Student>();
// Adding Students
for (Student s : studentData) {
System.out.println(s.rollNo + " " + s.name);
}
2. Removing Elements:
HashSet<String> carData = new HashSet<String>();
carData.remove("Volkswagen");
3. Handling Duplicates:
class Student {
// ... (equals() and hashCode() implementations)
}
HashSet<Student> studentData = new HashSet<Student>();
studentData.add(s1);
studentData.add(s2); // Ignored due to duplicate hashcode
studentData.add(s3);
4. Creating HashSet from a Collection:
ArrayList<Integer> list = new ArrayList<Integer>();
HashSet<Integer> hset = new HashSet<Integer>(list);
hset.add(5);
Hierarchy of HashSet Class
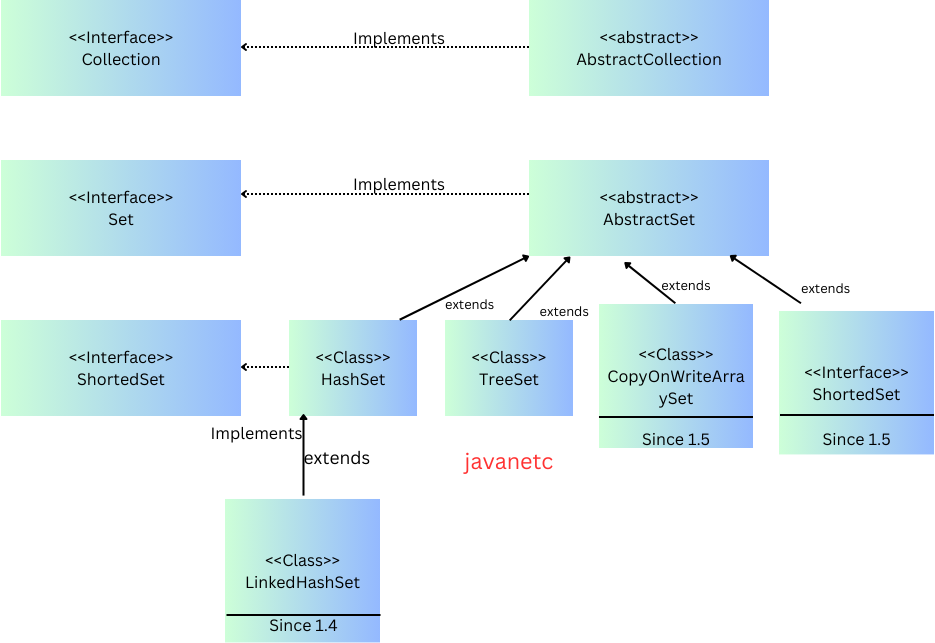
In Java, the HashSet class not only implements the Set interface but also extends the AbstractSet. The Set interface, in turn, is an extension of the Collection interface, which inherits from the Iterable interface.
Conclusion:
HashSet in Java is a powerful data structure in Java for scenarios requiring unique, unordered elements. Understanding its features and methods empowers developers to leverage its capabilities efficiently.