Introduction:
In the vast realm of Java programming, annotations in Java stand out as powerful metadata that enhance code readability, maintainability, and performance. This comprehensive guide will delve into the intricacies of annotations in Java, shedding light on what they are, why they matter, and how they can be effectively employed in your projects.
Table of Contents
What Are Annotations in Java?
Annotations, introduced in Java 5, are a form of metadata that provide information about the code to the compiler, runtime, or other tools. They can be added to declarations like classes, methods, fields, and parameters. This metadata enhances the understanding of the code and facilitates better communication between developers and tools.
Key Characteristics of Annotations in Java:
- @Retention: Annotations in Java can have different retention policies, specifying how long they should be retained. The three main retention policies are SOURCE, CLASS, and RUNTIME.
SOURCE
: Annotations are retained only in the source code and are ignored by the compiler.CLASS
: Annotations are retained during compilation but ignored by the Java Virtual Machine (JVM) at runtime.RUNTIME
: Annotations are retained both at compile time and runtime, making them accessible via reflection.
- @Target: This annotation defines where the annotation can be applied. Common targets include
TYPE
(class, interface),METHOD
,FIELD
,PARAMETER
, etc.
Why Annotations Matter in Java Development:
Annotations in Java is offer several advantages that contribute to cleaner code, improved development processes, and better tooling support.
- Code Organization and Readability: Annotations serve as a form of documentation, providing additional information about the code without cluttering the source files. This improves code readability and helps developers understand the purpose and usage of various elements.
- Compiler Checks and Warnings: Certain annotations, like
@Override
and@Deprecated
, trigger compiler checks and warnings. This helps catch errors and ensures adherence to coding standards. - Customization and Configuration: Annotations allow developers to add custom metadata to code, enabling configuration and customization. Frameworks like Spring heavily utilize annotations for dependency injection, transaction management, and more.
Predefined / Standard Annotations in Java:
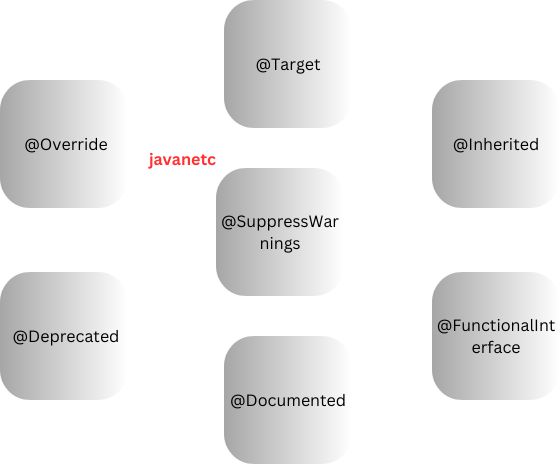
Exploring Commonly Used Annotations in Java:
1. @Override:
The @Override
annotation is used to indicate that a method in a subclass is intended to override a method declared in its superclass. This helps avoid accidental method name misspellings and ensures correct method overriding.
@Override
public void performAction()
2. @Deprecated:
The @Deprecated
annotation signals that a method, class, or field is deprecated and should not be used. This is crucial for maintaining codebases, as it informs developers about deprecated elements that may be removed in future releases.
@Deprecated
public class OldFeature {
// Deprecated class implementation
}
3. @SuppressWarnings:
The @SuppressWarnings
annotation is used to suppress specific compiler warnings that might be generated. This can be helpful in cases where developers are aware of potential issues and want to suppress certain warnings without affecting the entire codebase.
@SuppressWarnings("unchecked")
List<String> oldList = new ArrayList();
4. @FunctionalInterface:
Introduced in Java 8, the @FunctionalInterface
annotation ensures that an interface has only one abstract method, making it a functional interface. This is a key concept in the context of lambda expressions and the functional programming paradigm.
@FunctionalInterface
public interface Calculator {
int calculate(int a, int b);
}
Annotations in Action: Real-world Examples
1. Spring Framework:
The Spring Framework leverages annotations extensively for configuration and dependency injection. Annotations like @Autowired
, @Component
, and @RequestMapping
simplify the development of Java applications by reducing the need for XML configuration.
@Controller
@RequestMapping("/api")
public class MyController {
@Autowired
private DataService dataService;
@RequestMapping("/data")
public String fetchData() {
// Business logic using autowired dataService
return "result";
}
}
2. JUnit Testing:
Annotations play a crucial role in the JUnit testing framework. Annotations such as @Test
, @Before
, and @After
allow developers to define and organize test cases easily.
public class MyTest {
@Before
public void setUp() {
// Perform setup actions before each test execution
}
@Test
public void testAddition() {
// Test case logic
}
@After
public void tearDown() {
// Cleanup code executed after each test
}
}
Harnessing the Power of Annotations in Java for Improved Productivity:
1. Custom Annotations:
Developers can create their own annotations to capture domain-specific metadata. This is particularly useful in scenarios where standard annotations do not fully address the requirements of a specific project.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface LogExecutionTime {
// Additional elements can be defined
}
2. Using Reflection:
The java.lang.reflect
package allows developers to inspect and manipulate annotations at runtime. Reflection provides a powerful mechanism to access and utilize annotation information programmatically.
Method method = MyClass.class.getMethod("myAnnotatedMethod");
Annotation[] annotations = method.getAnnotations();
Difference Box: Annotations vs. Comments
Annotations and comments both provide a way to add information to the code, but they serve different purposes.
Aspect | Annotations | Comments |
---|---|---|
Purpose | Provide metadata and instructions to tools | Add human-readable explanations or notes |
Visibility | Available at compile-time and runtime | Only visible in the source code |
Effect on Execution | Can influence the behavior of the program | No impact on the program’s functionality |
Structured Information | Structured metadata with predefined roles | Free-form text with no predefined structure |
Conclusion:
Annotations in Java are a powerful tool that significantly enhances the expressiveness and maintainability of code. Whether it’s leveraging built-in annotations or creating custom ones, developers can harness the potential of annotations to streamline development processes, improve code quality, and enable seamless integration with various frameworks and tools.
Incorporating annotations in your Java projects empowers you to write cleaner, more organized code that is easier to understand and maintain. As you embark on your coding journey, embrace the versatility of annotations and unlock a new dimension of efficiency in Java development.