Introduction
Sockets facilitate the exchange of data between processes, whether they’re on the same machine or different machines within a network. They consist of an IP address and a software port number, enabling communication between various processes. Essentially, sockets provide the means to identify the destination application for data transmission through the combination of IP addresses and port numbers.
Table of Socket Programming in Computer Network
What is Socket Programming in Computer Networks?
Socket programming in computer network enables the exchange of data between processes running on the same or different machines. It forms the endpoint for bidirectional communication between two programs within a network.
Primarily employed in client-server architectures, sockets facilitate communication among multiple applications. Socket programming guides us on utilizing the socket API to establish communication between local and remote processes.
A socket is formed by combining the IP address and port number of the software. This amalgamation allows processes to determine the system address and the application’s address for data transmission. The IP address and port number are typically separated by a colon (:), for example, 192.168.1.67:80, 155.2.12.23:77, etc.
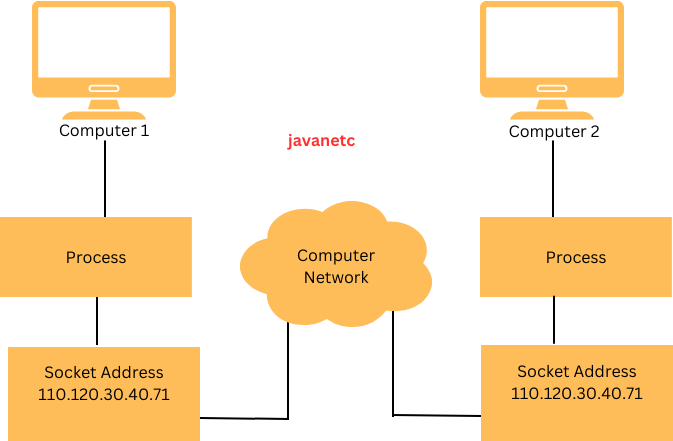
Socket Programming in Computer Network
Which Classes are Used for Connection-Less Socket Programming in Computer Network?
Connection-less Socket Programming in Computer Network operates without the need for connection establishment or termination, making it suitable for transmitting data over the network without prior setup. In this context:
- DatagramSocket and DatagramPacket classes are utilized.
- DatagramSocket represents a connectionless socket for sending and receiving datagram packets.
- DatagramPacket serves as a message container exchanged between communicating entities.
- Datagram packets may arrive in any order, regardless of their sending sequence.
These classes facilitate efficient communication in scenarios where connection establishment overhead is not desired, allowing for streamlined data transmission over the network.
Socket Programming in TCP
TCP, or Transmission Control Protocol, operates as a reliable, connection-oriented protocol within the transport layer. When engaging in TCP socket programming, the process unfolds as follows:
Client-side:
- Socket Creation: Begin by creating a socket using the
socket()
function. - Connection Establishment: Utilize the
connect()
function to establish a connection with the server address. - Data Transmission: Transmit data between the communicating parties through the
read()
andwrite()
functions. - Connection Termination: Upon completion of data transmission, close the connection using the
close()
function.
Server-side:
- Socket Establishment: Initiate a socket using the
socket()
function. - Binding to Address: Employ the
bind()
function to bind the socket to a specific address. - Listening for Connections: Use the
listen()
function to await client connections. - Connection Acceptance: Accept incoming client connections with the
accept()
function. - Data Transmission: Exchange data between the server and clients utilizing the
read()
andwrite()
functions.
By adhering to these steps, TCP socket programming facilitates robust, connection-oriented communication between networked entities.
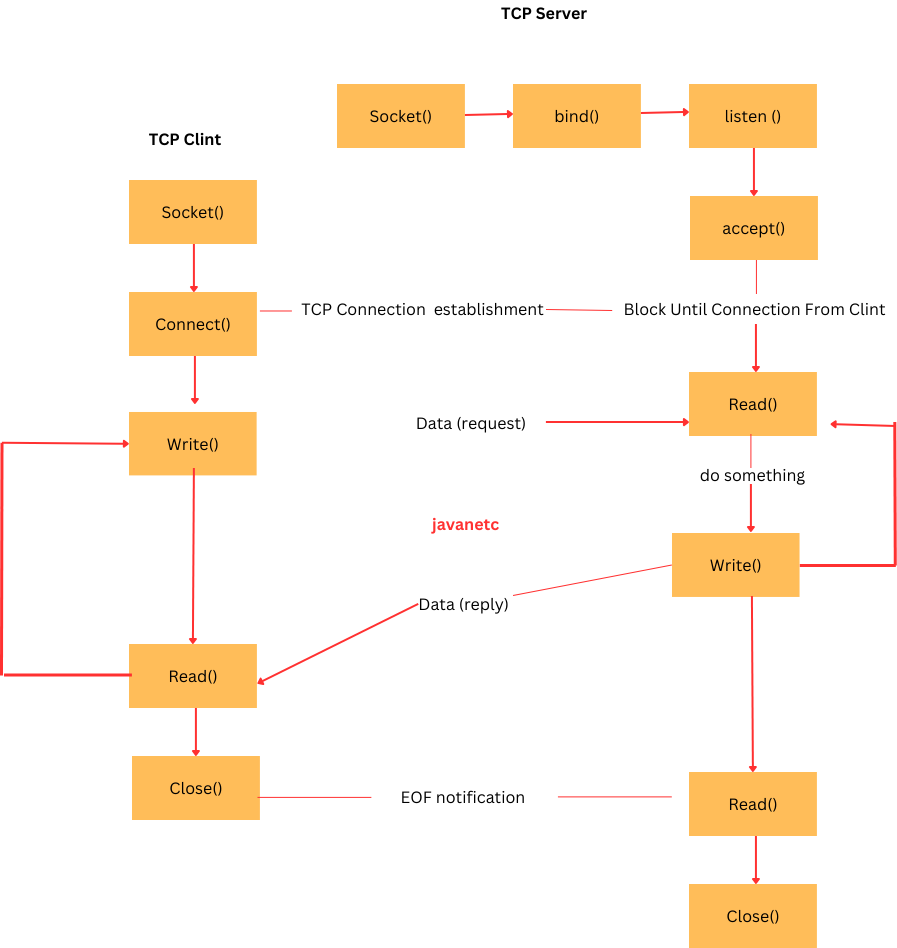
Socket Programming in UDP
UDP, or User Datagram Protocol, operates as a connection-less and unreliable protocol within the transport layer. Unlike TCP, UDP does not require connection establishment before transmitting data. Here’s a breakdown of establishing UDP socket connections:
Client-side:
- Socket Creation: Begin by creating a socket using the
socket()
function. - Data Transmission: Utilize the
sendto()
function to send data to the intended recipient, and userecvfrom()
to receive data from them.
Server-side:
- Socket Establishment: Create a socket using the
socket()
function. - Binding to Address: Employ the
bind()
function to bind the socket to a specific address. - Data Transmission: Exchange data using
recvfrom()
to receive data from clients andsendto()
to send data back to them.
By following these steps, UDP socket programming enables lightweight, connection-less communication between networked entities.
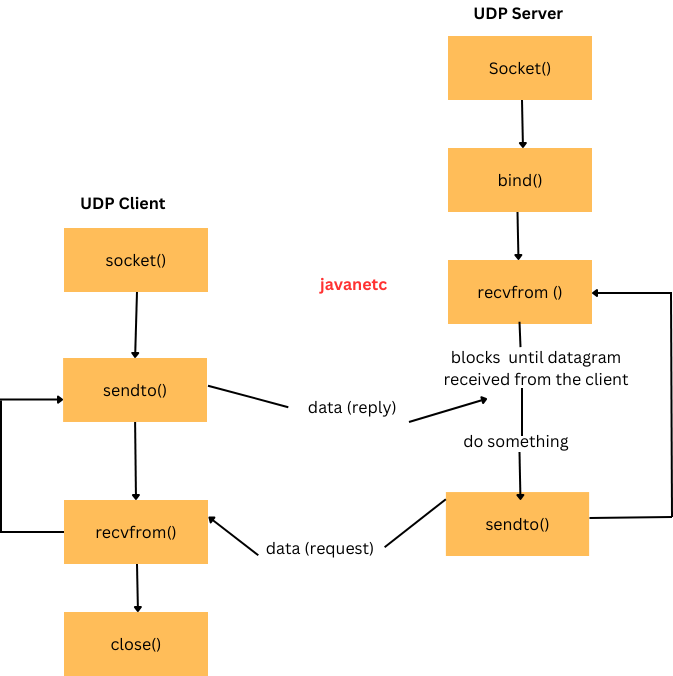
Socket Programming Interface Types
Socket programming interfaces come in three main types:
- Stream Sockets: These are the most common type, offering a connection-oriented service. Parties establish a socket connection before transmitting data, ensuring that data arrives in the order it was sent.
- Datagram Sockets: Providing connection-less services, datagram sockets do not require prior connection establishment. Parties transmit datagrams as needed, with data potentially being lost or arriving out of order. Despite these limitations, datagram sockets offer greater flexibility compared to stream sockets.
- Raw Sockets: Raw sockets bypass the built-in support for standard protocols like UDP and TCP, enabling developers to implement custom low-level protocols. This interface is useful for specialized networking tasks requiring fine-grained control over packet transmission and reception.
These socket programming interfaces cater to various networking requirements, empowering developers to tailor their applications to specific use cases.
Conclusion
A socket comprises an IP address and a software port address, enabling communication between processes. For connection-oriented socket programming, the Socket and ServerSocket classes are utilized, while DatagramSocket and DatagramPacket classes are employed for connectionless socket programming.
UDP operates as a connection-less, unreliable protocol within the transport layer, while TCP functions as a connection-oriented, reliable protocol.
The three main types of Socket Programming in Computer Network interfaces are Stream sockets, Datagram sockets, and raw sockets.
In TCP socket programming on the client-side, functions such as socket(), connect(), read(), write(), and close() are commonly used. On the server-side, functions like socket(), bind(), listen(), read(), write(), and accept() are utilized.
For UDP socket programming on the client-side, functions such as socket(), recvfrom(), and sendto() are commonly used. On the server-side, functions like socket(), recvfrom(), sendto(), and bind() are employed.