Introduction:
In the realm of Java programming, effective management of date and time has always been a critical aspect of application development. With the introduction of the java.time API, developers now have a robust and comprehensive toolkit for handling date and time-related operations. In this blog post, we’ll delve into the various classes and methods provided by the java.time API, uncovering their functionalities and demonstrating how they can be effectively employed in your Java applications.
Table of Contents
Understanding the java.time API:
The java.time API was introduced in Java 8 to address the limitations and complexities of the previous java.util.Date and java.util.Calendar classes. It is part of the java.time package and includes a set of classes for representing dates, times, durations, and periods. Let’s explore some of the key classes and their methods.
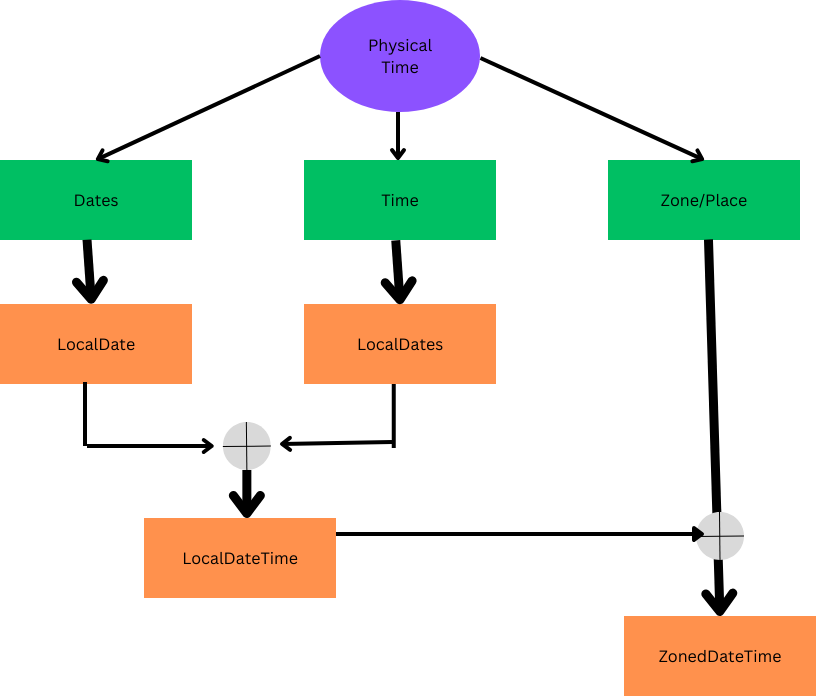
1. LocalDate Class:
The LocalDate class represents a date without a time component. It is widely used for tasks where only the date is relevant, such as birthday calculations or date-based comparisons.
Key Methods:
now()
: Obtains the current date from the system clock.plusDays(long daysToAdd)
: Adds the specified number of days to the current date.minusMonths(long monthsToSubtract)
: Subtracts the specified number of months from the current date.
2. LocalTime Class:
The LocalTime class represents a time without a date component. It’s useful for scenarios where only the time of day matters, such as scheduling events or tracking elapsed time.
Key Methods:
- Create a
LocalTime
instance with the specified hour and minute using theof
method:of(int hour, int minute)
. minusHours(long hoursToSubtract)
: Subtracts the specified number of hours from the current time.truncatedTo(ChronoUnit unit)
: Truncates the time to the specified precision, such as minutes or seconds.
3. LocalDateTime Class:
Combining the features of LocalDate and LocalTime, the LocalDateTime class represents a date and time without considering time zones. It’s a versatile class for general-purpose date and time handling.
Key Methods:
atZone(ZoneId zone)
: Converts this date-time to aZonedDateTime
with a specified time zone.plus(Duration amountToAdd)
: Adds a duration to the date-time.
4. ZonedDateTime Class:
The ZonedDateTime class extends LocalDateTime to include time zone information. It’s crucial when dealing with global applications or scenarios requiring precise time zone-aware calculations.
Key Methods:
withZoneSameInstant(ZoneId zone):
Returns a copy of thisZonedDateTime
with a different time zone, maintaining the same instant.
Why Choose java.time API?
The java.time API offers several advantages over its predecessors:
Immutable Classes: All classes in the java.time API are immutable, ensuring thread-safety and eliminating side effects.
Clarity and Simplicity: The API provides clear and concise classes, making code more readable and reducing the likelihood of errors.
Enhanced Functionality: With features like support for ISO-8601 standards, improved handling of daylight saving time, and comprehensive formatting options, the java.time API outshines its predecessors.
Examples:
Let’s illustrate the power of the java.time API with a few examples.
Example 1: Calculating Age
LocalDate birthDate = LocalDate.of(1990, 5, 15);
LocalDate currentDate = LocalDate.now();
long years = ChronoUnit.YEARS.between(birthDate, currentDate);
long months = ChronoUnit.MONTHS.between(birthDate, currentDate) % 12;
System.out.println("Age: " + years + " years, " + months + " months");
Example 2: Meeting Scheduler
LocalDateTime meetingDateTime = LocalDateTime.of(2023, 12, 15, 14, 30);
ZoneId newYorkTimeZone = ZoneId.of("America/New_York");
ZonedDateTime newYorkMeetingTime = meetingDateTime.atZone(newYorkTimeZone);
System.out.println("Meeting time in New York: " + newYorkMeetingTime);
Differences between java.time and java.util.Date:
Let’s highlight some key differences between the java.time
API and the older java.util.Date
class.
Feature | java.time API | java.util.Date |
---|---|---|
Immutability | Immutable | Mutable |
Thread Safety | Thread-safe | Not thread-safe |
Time Zone Handling | Yes (ZonedDateTime) | Limited |
Readability | Clear and concise | Verbosity and ambiguity |
java.time
API and the older java.util.Date
classConclusion:
In conclusion, the java.time API revolutionizes date and time handling in Java applications. Its elegant classes and powerful methods simplify complex operations, providing developers with a reliable toolkit. Whether you’re calculating age, scheduling meetings, or dealing with global time zones, the java.time API has you covered. Make the switch today for cleaner, safer, and more efficient date and time management in your Java projects.
Feel free to explore the official documentation for more in-depth information on the java.time API. Happy coding!